In this tutorial, we will discuss on how to create the loops in C with the help of code explanation. Also, we will learn the three types of loops in C such as while loop, do – while loop, and for loop.
What are loops in C?
In C program, a loop is being used for the execution of a block of code at a certain time according to the given statement in a loop. It means it will execute the same code into a multiple times to save the code. In addition, it will help to pass through the elements of an array.
The Three Types of Loops in C
- while loop
- do – while loop
- for loop
The while loop in C
In C program, a while loop will execute the code until the statement is false.
Syntax used in while loop:
while(statement){
//The condition of the code
}
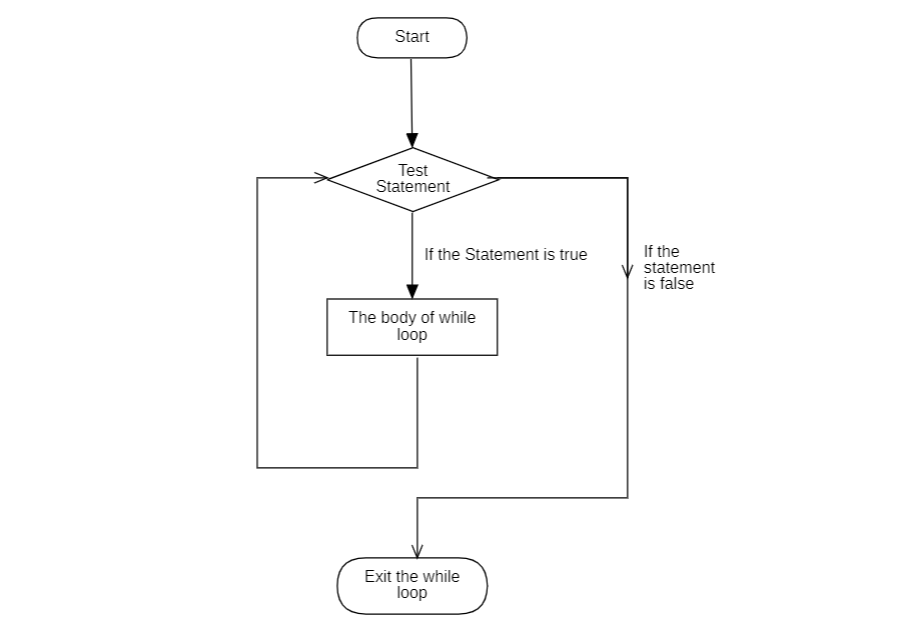
For the illustration above, when the statement is tested and the result is false, the loop body will be skipped and it will direct to the exit while the statement is tested and the result is true the loop will be executed.
Example of while loop in C
#include <stdio.h> int main () { /* local variable definition */ int i = 1; /* while loop execution */ while( i < 10 ) { printf("The value of i is: %d\n", i); i++; } return 0; }
Output:
The value of i is: 1 The value of i is: 2 The value of i is: 3 The value of i is: 4 The value of i is: 5 The value of i is: 6 The value of i is: 7 The value of i is: 8 The value of i is: 9
The for Loop in C
In C language, the for loop is a repeating structure that allows a loop to execute a specific number of times.
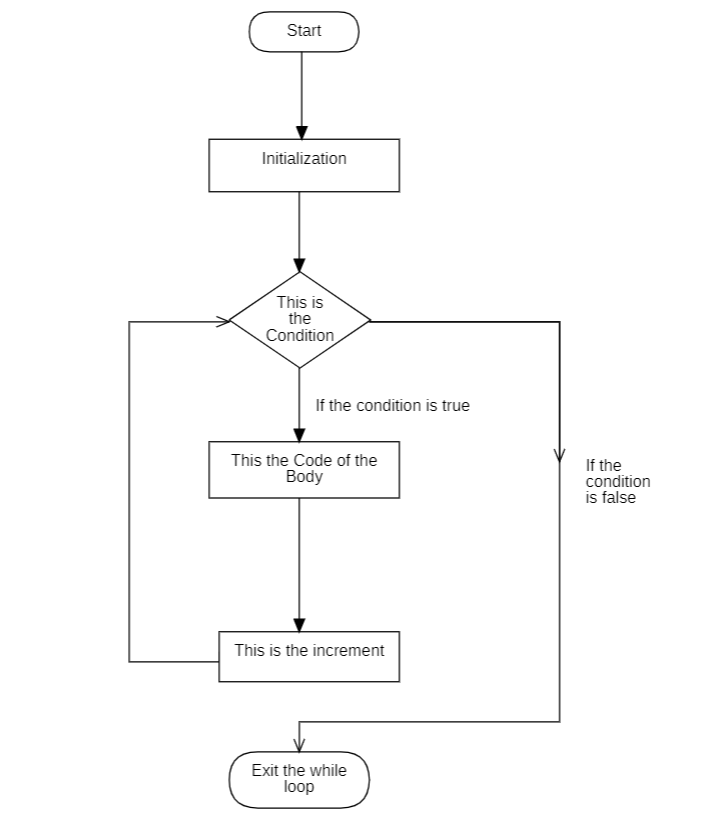
Syntax used in for loop in C
This is the syntax we will use in for loop.
for ( init; condition; increment ) {
condiiton(s);
}
How the for loops in C works?
- Firstly, the init will be executed first, and it is only once. It allows to declare and initialized any loop control variables. We cannot be required to put a condition, as long as that semicolon will appear.
- Secondly, the condition will be evaluated. If the condition is true, the body of the loop will be executed. While, If the condition is false, the body of the loop cannot be executed and the flow of control will jump to the next condition after the ‘for’ loop.
- Thirdly, the body of ‘for’ loop will be executed. The flow of control will back up to the increment condition. We can update any loop control variables by using this statement.
- Finally, the condition will be evaluated again. If the condition is true, the loop executes and it will process itself (body of loop, then increment step, and then again condition). If the statement is false, the ‘for’ loop will end.
Here is the example of for loops in C:
#include <stdio.h>
int main() {
int x;
for (x = 1; x < 6; ++x)
{
printf("%d ", x);
}
return 0;
}
Output:
1 2 3 4 5
Here is the explanation of the code example above.
- x will be initialized to 1.
- The test statement
x <
6 is evaluated. Then, 1 less than 6 the result is true. When the body offor
loop is executed. It will print the 1 or value of x on the screen window. - The update condition
++
x is executed. Now, the value of x will be 2. Again, the test statement is tested to true, and the body offor
loop will be executed. This will print 2 (value of x) on the screen window. - In the update statement
++
x is executed and the test statementx <
6 is tested. This process will go on until x will becomes 6. - If x becomes 6, x < 6 will be false, and the
for
loop ends.
The Do While Loop in C
In C program, the do-while loop is the same as a while loop, except for the fact that at least one execution is secured.
Here is the syntax of do while loop in C:
do{
//It will execute the code
}while(statement);
If the conditional statement appears at the end of the loop. Then the statement in the loop will execute once if the condition is tested.
If the statement is true, the flow of control will back up to do, and the conditions in the loop execute again. In this process will repeat until the given statement becomes false.
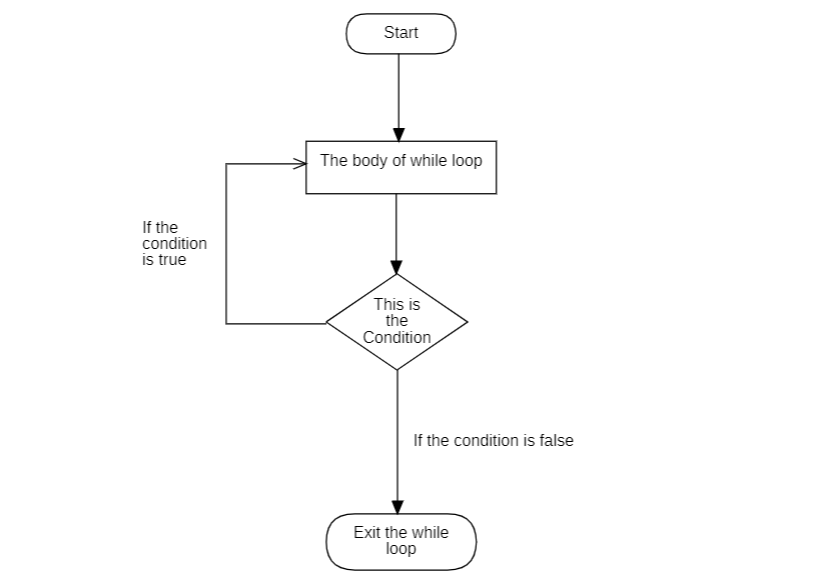
For example:
#include <stdio.h>
int main () {
/* Its the local variable definition */
int x = 30;
/* Its the do loop execution */
do {
printf("value of x: %d\n", x);
x = x + 1;
}while( x < 41 );
return 0;
}
Output:
value of x: 30
value of x: 31
value of x: 32
value of x: 33
value of x: 34
value of x: 35
value of x: 36
value of x: 37
value of x: 38
value of x: 39
value of x: 40
Loop Control Statements in C
In C programming language , a loop control statements can change the execution from its normal sequence. If the execution leaves a scope, all the automated objects that were created in that capacity are destroyed.
- break statement – This is used to terminate the loop or switch statement and transfers the execution to the condition immediately following the loop or switch.
- continue statement – It can skip several statements according to the given condition.
- goto statement – It can provide the control to the labeled statement.
Conclusion
In conclusion, we have already learned and discussed all the loops in C and also we give the flow diagram of each loop.
We hope through this tutorial you could improve your skills loops in C and learn how we can use it for your software development projects.