What is PHP syntax?
PHP Syntax is a structure that defines PHP computer language, and it refers to the set of rules that define how PHP code is written and interpreted.
This PHP language looks familiar because the PHP syntax is based on C, Java, and Perl.
Example of PHP Syntax
Here’s the basic syntax of PHP code:
<?php
//create your php code here
?>
Here’s the Basic Example of PHP Syntax:
- PHP code must start with <?php “Opening tags” and end with ?>“Closing Tags“.
- PHP statements must end with a semicolon (;).
- Saving a PHP code the extension must end on “ .php“.
- This contains some PHP code and HTML tags.
What is Escaping to PHP?
The PHP parsing engine needs a way to distinguish PHP code from other elements on the page.
The mechanism for this and the code inside is called “Escape to PHP“
The four traditional ways a parser identifies PHP code are quite different:
- PHP Canonical Tags
- SGML-style (Short-open) tags
- ASP Style tags
- HTML script tags
PHP Canonical Tags
The opening tag of the PHP script is <?php and the closing tag is ?> called “Canonical Tags“.
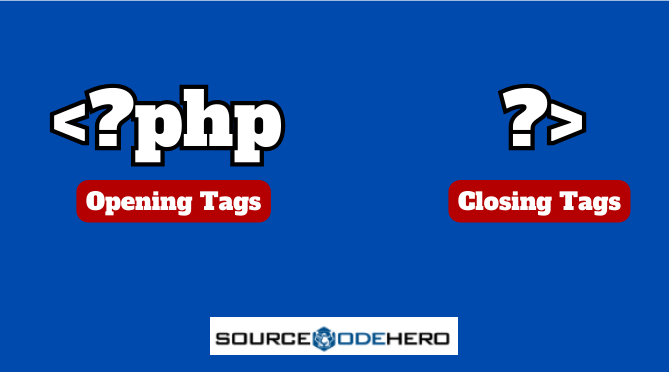
Example:
<?php
// this function is to print using Canonical tags
echo " Welcome to Source Code Hero" ;
?>
Output:
Welcome to Source Code Hero
PHP SGML-Style (Short Open) Tags
The PHP SGML-Style (Short Open) Tags are the shortest and easiest way to present PHP code, the Opening tag of SGML-Style is “<?” and the end is “?>“.
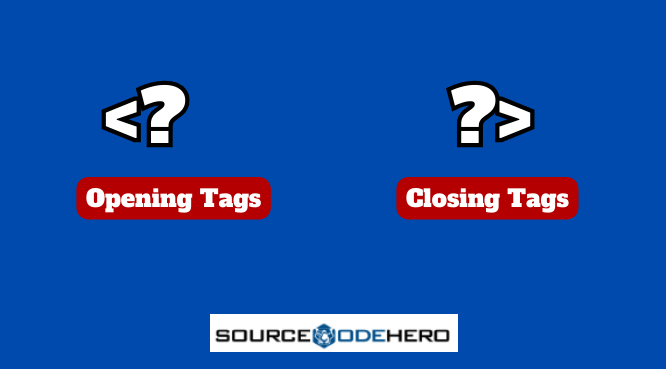
However, SGML-style tags are more difficult to configure than the canonical option, which is almost the default.
As you might expect, short tags are the shortest choice. For PHP to recognize tags, you must do one of two things.
- When configuring PHP, select the “Enable short tags” configuration option.
- Enable the short open tag setting in the php.ini file.
To parse XML with PHP, this option must be disabled because XML tags use the same syntax.
Example:
<?
// This function is to print using the SGML-style tags
echo "Sample of SGML-style Tags!"
?>
Short open tags start with “<?” and end with “?>”. Short style tags are only available if enabled in the php.ini configuration file on servers.
Output:
Sample of SGML-style Tags!
PHP ASP Style Tags
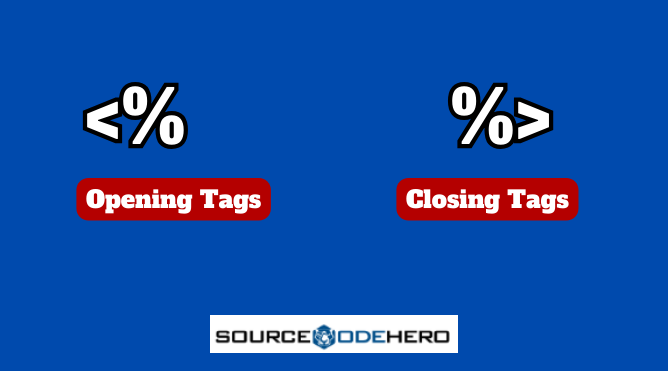
ASP-style tags mimic the tags used by Active Server Pages to identify blocks of code.
Example:
<%
// This tag can be used if you allow php.ini to use %
echo "Basic code of ASP style tags!!"
%>
For this code block to work, we need to edit the php.ini file.
Output:
Basic code of ASP style tags!!
PHP HTML Script Tags?
HTML is the first thing most website builders learn and most programmers and web developers, especially when integrating scripts from other files such as JavaScript or CSS.
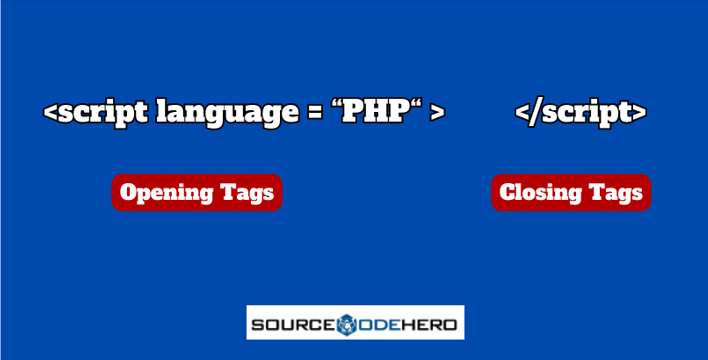
Here is the latest PHP code Example to construct PHP HTML Script Tags:
Filename: index.php
<!DOCTYPE html>
<html>
<head>
<title>PHP HTML Script Tags</title>
</head>
<body>
<?php
//this function is to print using the PHP HTML Script Tags.
echo "Sample PHP code using PHP HTML Script Tags";
?>
</body>
</html>
Output:
Sample PHP code using PHP HTML Script Tags
Although the specific tags option in PHP 7 is no longer used, other ways to handle HTML within PHP still exist.
Common approaches include:
- Embedding PHP code within HTML:
While generally discouraged due to the separation of concerns, you can still use < ?php … ? > within HTML. - Including external JavaScript:
For dynamic scripting, use tags to link to separate JS files - Separate templates:
Create distinct .html and .php files, with PHP generating the HTML content dynamically.
Understanding Case Sensitivity in PHP
Knowing how Case Sensitivity works in PHP is crucial for writing bug-free and consistent code.
Here’s the explanation about the case sensitivity below.
PHP is insensitive to whitespace
This includes tabs, spaces, and carriage returns that are not visible on the screen.
A space corresponds to any number of spaces or carriage returns.
This means PHP will ignore any spaces, tabs, carriage returns, or carriage returns on more than one line.
If PHP doesn’t recognize the semicolon, it treats multiple lines as a single command.
Example:
<?php
// Assigning value to a variable with different amounts of whitespace:
$message1 = "Welcome to "; // tabs space after assignment operator
$message2 =
" Source code hero! "; // Multiple spaces before and after value
$message3 = "Have a Great Day!! "; // Tabs and spaces mixed
// All three lines achieve the same result: storing the string in the $message variable.
$message = $message1 . $message2 . $message3;
echo " $message"// Outputs "This is the same string with different formatting"
?>
Output:
Welcome to Source code hero! Have a Great Day!!
PHP is case-sensitive
Except for variables, all PHP keywords, functions, and class names (while, if, echo, else, etc.) are case-insensitive.
PHP is not entirely case-sensitive and it’s more accurate to say that it has mixed-case sensitivity depending on the element you’re dealing with and the only variables with different cases treated differently.
Example:
<?php
$name = "John Doe"; // Variable with lowercase name
echo "Hello, $name!"; // Outputs "Hello, John Doe!"
$NAMe = "Jane Doe"; // Different variable with uppercase name
// Incorrectly referencing the non-existent variable will cause an error here
echo "Hello, $NAME!"; // Outputs an error: "Undefined variable: NAME"
// Same string, different variables, different results due to case sensitivity
?>
Output:
Hello, John Doe!
PHP Warning: Undefined variable $NAME in C:\xampp\htdocs\Sample\index.php on line 8
Hello, !
Understanding Comments in PHP
Understanding comments in PHP is crucial for writing clean, maintainable, and collaborative code.
The purpose of a comment is to make the code easier to read and understand.
It is used to help other users and developers describe the code and its purpose.
When the code is reviewed after a while, the comments help the programmer remember what he is doing.
What are comments?
Comments are lines of code ignored by the PHP interpreter, are not executed, and serve as instructions and explanations for developers reading the code.
Types of comments:
- Single-line comments.
- Multi-line comments.
Single Line Comment
As the name suggests, these are single lines or short explanations, which you can add to your code.
Comments start with “//” or “#” and continue or extend at the end of the line.
Example:
<?php
// This is a single line comment
// These can't be extended to more lines
echo "Sample of PHP Single Line Comment."; // This is a comment also
# This is also a single line comment
?>
Output:
Sample of PHP Single Line Comment.
Multi-line or Multiple line Comment
Multiple line Comment allows multiple lines to be inserted into a single tag and can be expanded to as many lines as the user desires.
Start with “/” and end with “/”. Span multiple lines.
Example:
<?php
/*
This is a multi line or Multiple line comment
You can use this to comment a code or to describe the functions of the code
*/
echo "Sample of PHP Multiple Line Comment.";
?>
Output:
Sample of PHP Multiple Line Comment.
Understanding PHP Blocks
In PHP, a Block refers to a group of statements enclosed in curly braces {}, used to define a variety of variables, control structures, and functions/methods.
PHP block demonstrating a conditional statement:
Example:
<?php
// Define a variable
$age = 25;
// Check if the age meets a certain condition
if ($age >= 18) {
echo "You are eligible to vote."; // Code block executed if the condition is true
} else {
echo "You are not eligible to vote."; // Code block executed if the condition is false
}
?>
Output:
You are eligible to vote.
Conclusion
There you have it, we have covered the basic syntax of PHP. We have explained every detail and shown some examples for better understanding.
If you want to learn and understand PHP from the beginning, you should check the following article:
If you have questions or inquiries about the PHP Basic Syntax and Comments Overview, don’t hesitate to leave a comment below.
We’d love to hear it!